This page will help you get started with Publisher Performance API.
Welcome to Publisher Performance API. Using this API you will be able to grab the latest data from Appier's Publisher Portal.
API Documentation
Headers
- appier-client-id
- appier-secret-id
Params
- start_date
- end_date
- timezone
Endpoint
- https://pubdash.apx.appier.net/api/v1/performance/list?from={}&to={}&timezone={}
Usage Example
Please use your own appier-client-id and appier-secret-id found on Publisher Portal.
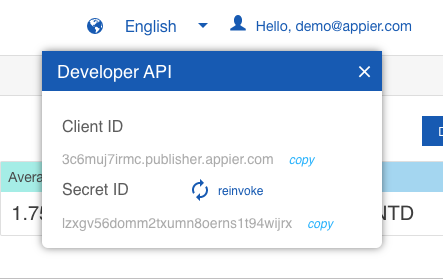
For demo purposes please use the following demo ids:
- appier-client-id: 3c6muj7irmc.publisher.appier.com
- appier-secret-id: lzxgv56domm2txumn8oerns1t94wijrx
import json
import urllib
import csv
import requests
headers = {
# Please fill out your credential (client id/secret id) info from publisher portal
'appier-client-id': '',
'appier-secret-id': ''
}
# Please fill out your date
start_date = '20171215'
end_date = '20171225'
# Please fill out your timezone
timezone = 'UTC%2B8'
res = requests.get('https://pubdash.apx.appier.net/api/v1/performance/list?from={}&to={}&timezone={}'.format(start_date, end_date, timezone), headers=headers)
result_data = json.loads(res.text)
print(result_data)
Output Example
{
"stat": {
"20200610": {
"6388": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6389": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6390": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6391": {
"click": 16,
"impression": 959,
"revenue": 172.62,
"traffic": 3296
},
"6392": {
"click": 19,
"impression": 915,
"revenue": 164.7,
"traffic": 3247
}
},
"20200611": {
"6388": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6389": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6390": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6391": {
"click": 0,
"impression": 3,
"revenue": 0.54,
"traffic": 4150
},
"6392": {
"click": 17,
"impression": 1295,
"revenue": 233.1,
"traffic": 4093
}
},
"20200612": {
"6388": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6389": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6390": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 0
},
"6391": {
"click": 0,
"impression": 0,
"revenue": 0,
"traffic": 4125
},
"6392": {
"click": 5,
"impression": 901,
"revenue": 162.18,
"traffic": 4220
}
}
},
"username": "鳳梨傳媒股份有限公司",
"zones": {
"6388": {
"currency": "NTD",
"description": "",
"height": 250,
"name": "6388_鳳梨傳媒股份有限公司_4gtv_PC_PD_PC_300x250 右上第一顆_PD",
"width": 300,
"zoneid": 6388
},
"6389": {
"currency": "NTD",
"description": "",
"height": 250,
"name": "6389_鳳梨傳媒股份有限公司_4gtv_PC_PD_PC_300x250 右第二顆_PD",
"width": 300,
"zoneid": 6389
},
"6390": {
"currency": "NTD",
"description": "",
"height": 600,
"name": "6390_鳳梨傳媒股份有限公司_4gtv_PC_PD_PC_300x600_PD",
"width": 300,
"zoneid": 6390
},
"6391": {
"currency": "NTD",
"description": "",
"height": 350,
"name": "6391_鳳梨傳媒股份有限公司_4gtv_All_Video_PD",
"width": 350,
"zoneid": 6391
},
"6392": {
"currency": "NTD",
"description": "",
"height": 350,
"name": "6392_鳳梨傳媒股份有限公司_4gtv_All_Video_PD",
"width": 350,
"zoneid": 6392
}
}
}